Hey Joan,
I finally was able to made the necessary changes
and it works like a charm
KiCAD_CopperFillPoly.py (1.6 KB)
import sys, os
import shutil
import math
LIST_pts = [" (polygon\n (pts\n (xy “,” (filled_polygon\n (pts\n (xy “,”) (xy “,”)\n )\n )"]
def FNC_poly (cntr, # (x,y)
innerRadius,
outerRadius,
sides,
startangle,
angle,
):
STR_data = ""
baseX = cntr[0]
baseY = cntr[1]
sideangle = angle / sides
for i in range(sides):
pointX = baseX + innerRadius * math.sin(math.radians(sideangle*(i+0.5) + startangle))
pointY = baseY + innerRadius * math.cos(math.radians(sideangle*(i+0.5) + startangle))
STR_data += "{:.4f}".format(pointX) + " " + "{:.4f}".format(pointY) + LIST_pts[2]
for i in range(sides):
pointX = baseX + outerRadius * math.sin(math.radians(sideangle*((sides-i-1)+0.5) + startangle))
pointY = baseY + outerRadius * math.cos(math.radians(sideangle*((sides-i-1)+0.5) + startangle))
STR_data += "{:.4f}".format(pointX) + " " + "{:.4f}".format(pointY) + LIST_pts[2]
STR_data = STR_data[:-6] + LIST_pts[3]
return LIST_pts[0] + STR_data + "\n" + LIST_pts[1] + STR_data
if name == ‘main’:
Center = [100.0,100.0] # x/y coordinates of the centre of the pcb sheet
innerRadius = 6 # mm
outerRadius = 7.5 # mm
Sides = 20
StartAngle = 75.0 # degrees
Angle = 30.0 # degrees
print FNC_poly (Center,
innerRadius,
outerRadius,
Sides,
StartAngle,
Angle,
)
thank your for your help!
I also want to provide a short how to for other people using this script.
1. open the script with the editor of your choice and change the parameters innerRadius, outerRadius, Sides, StartAngle and Angle to your needs. Save it!
2. under windows, navigate to the folder where the python script is stored and use the command: yourPythonScriptName.py > yourDesiredOutputFile.txt
to get the output data stored in a text file
3. open your PCB file and draw a simple polygon (shape doesn’t matter here) save it and close it
4. open your .kicad_pcb file with an editor and detect the polygon you’ve drawn earlier, this looks something like this:
(zone (net 1) (net_name +3V3) (layer F.Cu) (tstamp 0) (hatch edge 0.508)
(connect_pads (clearance 0.2))
(min_thickness 0.254)
(fill yes (arc_segments 32) (thermal_gap 0.508) (thermal_bridge_width 0.508))
(polygon
(pts
(xy 105.8154 101.4769) (xy 105.8521 101.3242) (xy 105.8847 101.1705) (xy 105.9133 101.0161) (xy 105.9379 100.8610) (xy 105.9584 100.7052) (xy 105.9748 100.5490) (xy 105.9872 100.3924) (xy 105.9954 100.2356) (xy 105.9995 100.0785) (xy 105.9995 99.9215) (xy 105.9954 99.7644) (xy 105.9872 99.6076) (xy 105.9748 99.4510) (xy 105.9584 99.2948) (xy 105.9379 99.1390) (xy 105.9133 98.9839) (xy 105.8847 98.8295) (xy 105.8521 98.6758) (xy 105.8154 98.5231) (xy 107.2692 98.1539) (xy 107.3151 98.3448) (xy 107.3559 98.5368) (xy 107.3917 98.7299) (xy 107.4224 98.9238) (xy 107.4480 99.1185) (xy 107.4685 99.3137) (xy 107.4839 99.5095) (xy 107.4942 99.7056) (xy 107.4994 99.9018) (xy 107.4994 100.0982) (xy 107.4942 100.2944) (xy 107.4839 100.4905) (xy 107.4685 100.6863) (xy 107.4480 100.8815) (xy 107.4224 101.0762) (xy 107.3917 101.2701) (xy 107.3559 101.4632) (xy 107.3151 101.6552) (xy 107.2692 101.8461)
)
)
)
5. open the output text file of the script and copy the section (pts…) looks something like this:
(pts
(xy 105.8154 101.4769) (xy 105.8521 101.3242) (xy 105.8847 101.1705) (xy 105.9133 101.0161) (xy 105.9379 100.8610) (xy 105.9584 100.7052) (xy 105.9748 100.5490) (xy 105.9872 100.3924) (xy 105.9954 100.2356) (xy 105.9995 100.0785) (xy 105.9995 99.9215) (xy 105.9954 99.7644) (xy 105.9872 99.6076) (xy 105.9748 99.4510) (xy 105.9584 99.2948) (xy 105.9379 99.1390) (xy 105.9133 98.9839) (xy 105.8847 98.8295) (xy 105.8521 98.6758) (xy 105.8154 98.5231) (xy 107.2692 98.1539) (xy 107.3151 98.3448) (xy 107.3559 98.5368) (xy 107.3917 98.7299) (xy 107.4224 98.9238) (xy 107.4480 99.1185) (xy 107.4685 99.3137) (xy 107.4839 99.5095) (xy 107.4942 99.7056) (xy 107.4994 99.9018) (xy 107.4994 100.0982) (xy 107.4942 100.2944) (xy 107.4839 100.4905) (xy 107.4685 100.6863) (xy 107.4480 100.8815) (xy 107.4224 101.0762) (xy 107.3917 101.2701) (xy 107.3559 101.4632) (xy 107.3151 101.6552) (xy 107.2692 101.8461)
)
6. go to your kicad_pcb file and replace the coordinates. save it.
7. done! if you open kicad again your polygon should look similar to this:
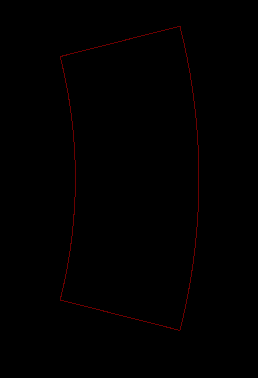
as mentioned earlier in this thread you need to connect or place a component inside the polygon to be able to fill it.
All credits here goes to @Joan_Sparky for making this cool script.